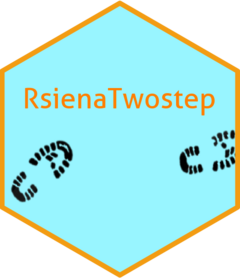
Introduction_RsienaTwoStep
Source:vignettes/Introduction_RsienaTwoStep.Rmd
Introduction_RsienaTwoStep.Rmd
rm(list=ls())
library(RsienaTwoStep)
#> Loading required package: foreach
1. Data sets
Let us have a look at the build-in data sets of
RsienaTwoStep
.
1.1. ts_net1
1.1.1. Adjacency matrix
ts_net1
#> [,1] [,2] [,3] [,4] [,5] [,6] [,7] [,8] [,9] [,10]
#> [1,] 0 0 0 0 0 0 0 0 0 0
#> [2,] 0 0 1 0 0 0 0 0 0 0
#> [3,] 1 0 0 0 0 1 0 0 0 0
#> [4,] 0 0 0 0 0 0 0 0 1 0
#> [5,] 0 0 0 0 0 0 0 0 0 0
#> [6,] 0 0 0 0 0 0 0 0 1 1
#> [7,] 1 0 0 0 0 0 0 0 0 0
#> [8,] 0 0 0 0 0 0 0 0 0 1
#> [9,] 0 0 0 1 0 0 0 1 0 0
#> [10,] 0 0 0 0 0 0 0 1 1 0
1.1.2. Plot
net1g <- igraph::graph_from_adjacency_matrix(ts_net1, mode="directed")
par(mar=c(.1, .1, .1, .1))
igraph::plot.igraph(net1g)
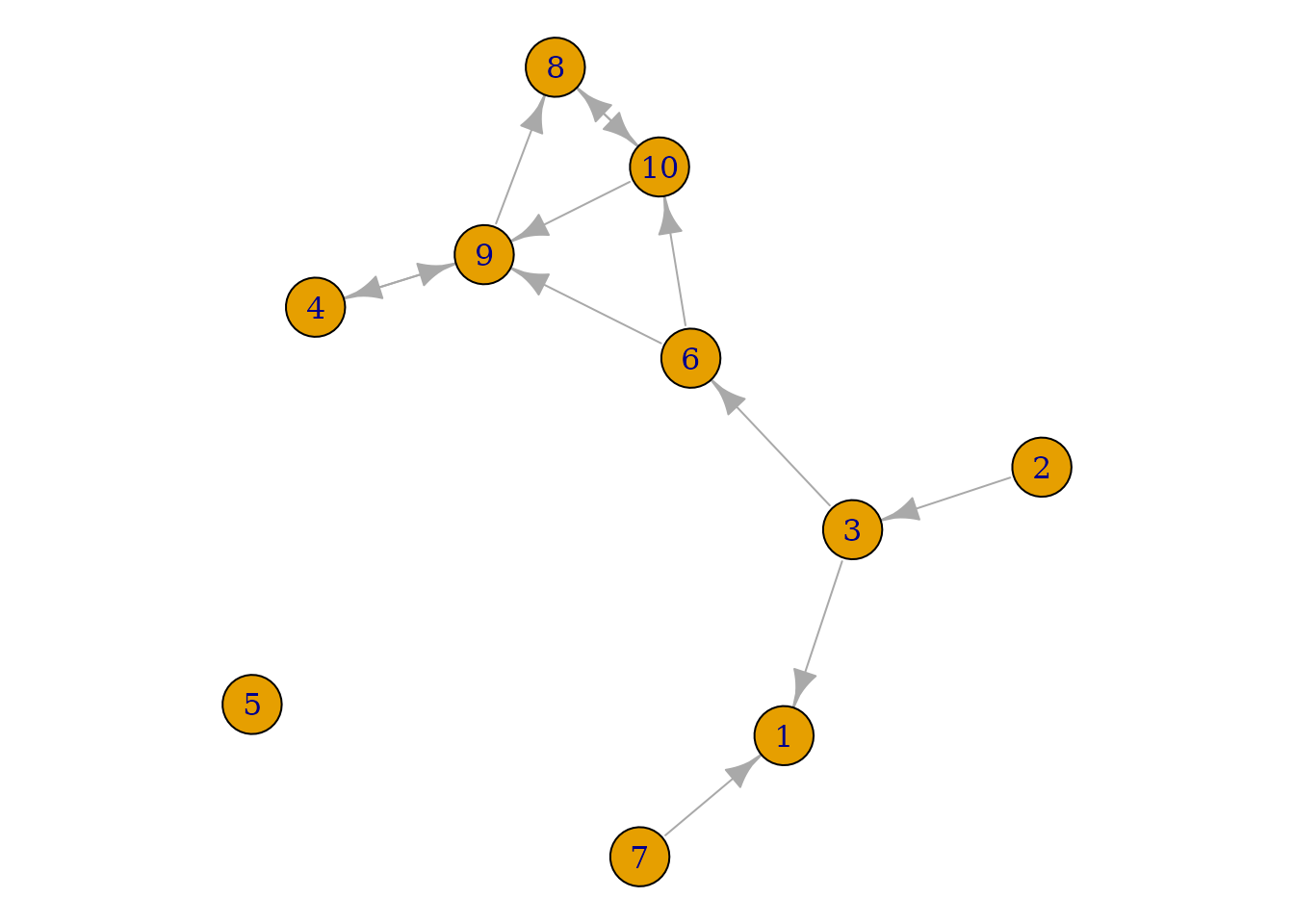
Figure 1.1.2. ts_net1
1.2. ts_net2
1.2.1. Adjacency matrix
ts_net2
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 1
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
1.2.2. Plot
net2g <- igraph::graph_from_adjacency_matrix(ts_net2, mode="directed")
par(mar=c(.1, .1, .1, .1))
igraph::plot.igraph(net2g)
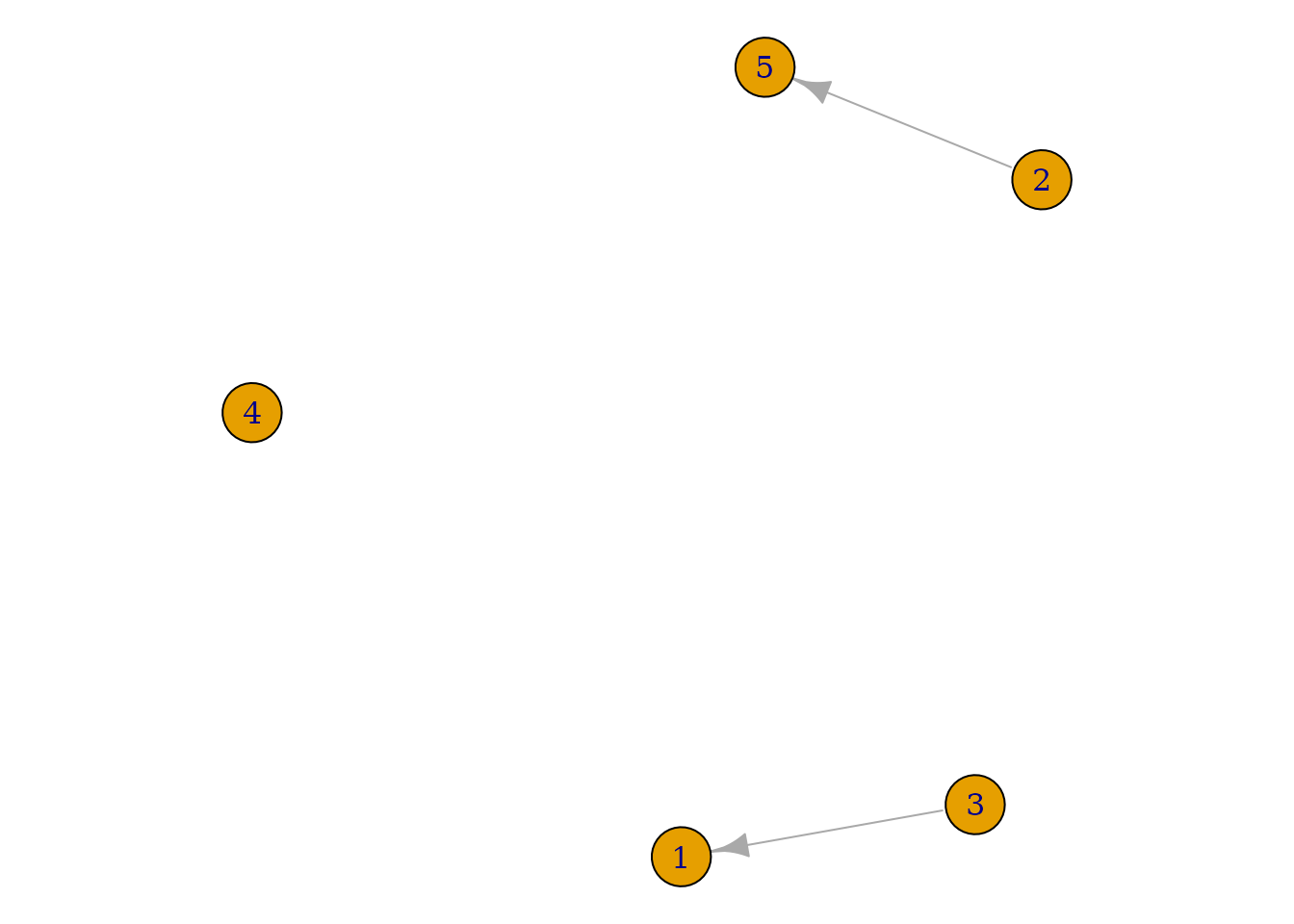
Figure 1.2.2. ts_net2
2. ABM ministep
2.1. Logic
- sample ego
- construct possible alternative future networks based on all possible
ministeps of ego
- calculate how sampled ego evaluates these possible networks
- Let the ego pick a network, that is, let agent decide on a
tie-change
- GOTO 1 (STOPPING RULE: until you think we have made enough ministeps)
2.2. Possible networks after ministep
Let us suppose we want to know what the possible networks are after
all possible ministeps of one actor part of ts_net2
. That
is, let us assume that it is ego#2’s turn to decide on tie-change. What
are the possible networks?
options <- ts_alternatives_ministep(net=ts_net2, ego=2)
options
#> [[1]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 1 0 0 0 1
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[2]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 1
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[3]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 1 0 1
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[4]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 1 1
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[5]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
ts_alternatives_ministep()
returns a list of all
possible networks after all possible tie-changes available to ego#2
given network ts_net2
. If you look closely you will see
that options[[2]]
equals the original network (i.e. ego#2
decided not to change any tie).
2.3. Network statistics
Which option will ego#2 choose? Naturally this will depend on which network characteristics (or statistics) ego#2 finds relevant. Let us suppose that ego#2 bases its decision solely on the number of ties it sends to others and the number of reciprocated ties it has with others.
Let us count the number of ties ego#2 sends to alters.
ts_degree(net=options[[1]], ego=2)
#> [1] 2
And in the second (original) option:
ts_degree(net=options[[2]], ego=2)
#> [1] 1
In the package RsienaTwoStep
there are functions for the
following network statistics
:
- degree:
ts_degree()
- reciprocity:
ts_recip()
- outdegree activity:
ts_outAct()
- indegree activity:
ts_inAct()
- outdegree popularity:
ts_outPop()
- indegree popularity:
ts_inPop()
- transitivity:
ts_transTrip()
- mediated transitivity:
ts_transMedTrip()
- transitive reciprocated triplets:
ts_transRecTrip()
- number of three-cycles:
ts_cycle3()
See Ripley et al. (2022) (Chapter 12) for the mathematical formulation. Naturally, you are free to define your own network statistics.
2.4. Evaluation function
But what evaluation value does ego#2 attach to these network
statistics and consequently to the network (in its vicinity) as a whole?
Well these are the parameters,
,
you will normally estimate with RSiena::siena07()
. Let us
suppose the importance for the statistic ‘degree’ is -1 and for the
statistic ‘reciprocity’ is 2.
So you could calculate the evaluation of the network saved in
options[[2]]
by hand:
with the evaluation function for agent . are the network statistics for network and the corresponding parameters (or importance).
1*-1 + 0*2
#> [1] -1
or with a little help of the network statistic functions:
Or you could use the ts_eval()
.
eval <- ts_eval(net=options[[2]], ego=2, statistics=list(ts_degree, ts_recip), parameters=c(-1,2))
eval
#> [1] -1
Now, let us calculate the evaluation of all 5 possible networks:
2.5. Choice function
So which option will ego#2 choose? Naturally this will be a stochastic process. But we see the last option has the highest evaluation. We use McFadden’s choice function (for more information see wiki), that is let be the probability that ego chooses network/alternative . The choice function is then given by:
with a vector of the value of each network statistics for network and is the vector of parameter values. Hence, is the value of the evaluation for network .
Let us force ego#2 to make a decision.
choice <- sample(1:length(eval), size=1, prob=exp(eval)/sum(exp(eval)))
print("choice:")
choice
print("network:")
options[[choice]]
#> [1] "choice:"
#> [1] 5
#> [1] "network:"
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 1 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
If we repeat this process, that is…:
- sample agent
- construct possible alternative networks
- calculate how sampled agent evaluates the possible networks
- Let the agent pick a network, that is, let agent decide on a
tie-change
- GO BACK TO 1 (STOPPING RULE: until you think we have made enough ministeps)
…we have an agent based model.
2.6. Stopping rule
But how many ministeps do we allow? Well, normally this is estimated
by siena07
by the rate
parameter. If we do not
make this rate parameter conditional on actor covariates or on network
characteristics, the rate parameter can be interpreted as the average
number of ministeps each actor in the network is allowed to make before
time is up. Let us suppose the rate
parameter is 2 . Thus
in total the number of possible ministeps will be
nrow(ts_net2)*rate
: 10. For a more detailed - and
more correct - interpretation of the rate parameter in
siena07
see: www.jochemtolsma.nl.
2.7. Example
To demonstrate the network evolution:
#in startvalues the first value refers to the rate parameter, the subsequent values to the statistics of statistics
ts_sims(startvalues = c(2,-1,2), net1=ts_net2, statistics=list(ts_degree, ts_recip),nsims=1, p2step=c(1,0,0), chain = TRUE )
#> [1] "nsim: 1"
#> [[1]]
#> [[1]]$final
#> [[1]]$final$net_n
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 1 0 0
#> [5,] 0 0 0 1 0
#>
#>
#> [[1]]$chain
#> [[1]]$chain$nets
#> [[1]]$chain$nets[[1]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 1
#> [3,] 0 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[2]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[3]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[4]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[5]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 1 0
#>
#> [[1]]$chain$nets[[6]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 1 0 0
#> [5,] 0 0 0 1 0
#>
#> [[1]]$chain$nets[[7]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 1 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 1 0 0
#> [5,] 0 0 0 1 0
#>
#> [[1]]$chain$nets[[8]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 1 0 0
#> [5,] 0 0 0 1 0
#>
#> [[1]]$chain$nets[[9]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 1 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[10]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 1 0 0 0
#> [2,] 0 0 0 0 0
#> [3,] 0 0 0 0 0
#> [4,] 0 0 1 0 0
#> [5,] 0 0 0 1 0
3. ABM twostep
3.1. Logic
The general logic of the ABM that allows for twosteps is very similar to the ABM ministep model:
- sample two agents
- construct possible alternative networks
- calculate how the sampled agents evaluate the possible
networks
- Let the agents together pick the subsequent network, that is, let
agents decide on the twostep (the simultaneous two ministeps)
- GOTO 1 (STOPPING RULE: until you think we have made enough ministeps/twosteps)
3.2. Sample two agents
-
Simultaneity: agents are sampled randomly
-
Strict coordination: only specific dyads are
sampled (with a specific distance between them, based on either out-,
in- or reciprocal ties)
- Weak coordination: agents are sampled randomly but only specific twosteps are regarded as ‘coordinated’ twosteps and, consequently only some of the possible alternative networks are included in the choice set for the dyad.
3.3. Possible networks after twostep
If we want to allow for simultaneity, we simply let first agent1 make all possible ministeps and then conditional on these alternative networks let agent2 make all possible ministeps. Please note that the order in which we let agents make the ministeps is not important. We simple construct all the networks that could result from agent1 and agent2 make a simultaneous ministep.
Exception: With weak coordination we will assess which possible alternative networks impact the evaluation function of both egos. Only those possible alternative networks are regarded as the result of coordination and included in the choice set. Thus, it is not necessarry for ego1 and ego2 to be connected at time1 but then they should at least be connected at time2 in such a way that they influence each others evaluation function.
The implementation in the current version ofRsieneTwoStep
is, however, a bit different. With weak coordination we simple assess the distance between ego1 and ego2 at time1 and time2. If at eithter time1 or time2 the distance is within the set threshold, we regard the twostep as a coordinated twostep.
3.4. Network statistics
We use the same network statistics as for the ABM ministep. But
please note, that not all existing network statistics of
RSiena
make sense. See for example the figure below.
Suppose the evaluation of the transitive triad for actor i
depends on whether path i to j was the closing path
(i.e., created after path h to j) or not. In a
twostep, both ties may be created simultaneously and we cannot
distinguish these two routes.
#> Warning: `layout.grid()` was deprecated in igraph 2.0.0.
#> ℹ Please use `layout_on_grid()` instead.
#> This warning is displayed once every 8 hours.
#> Call `lifecycle::last_lifecycle_warnings()` to see where this warning was
#> generated.
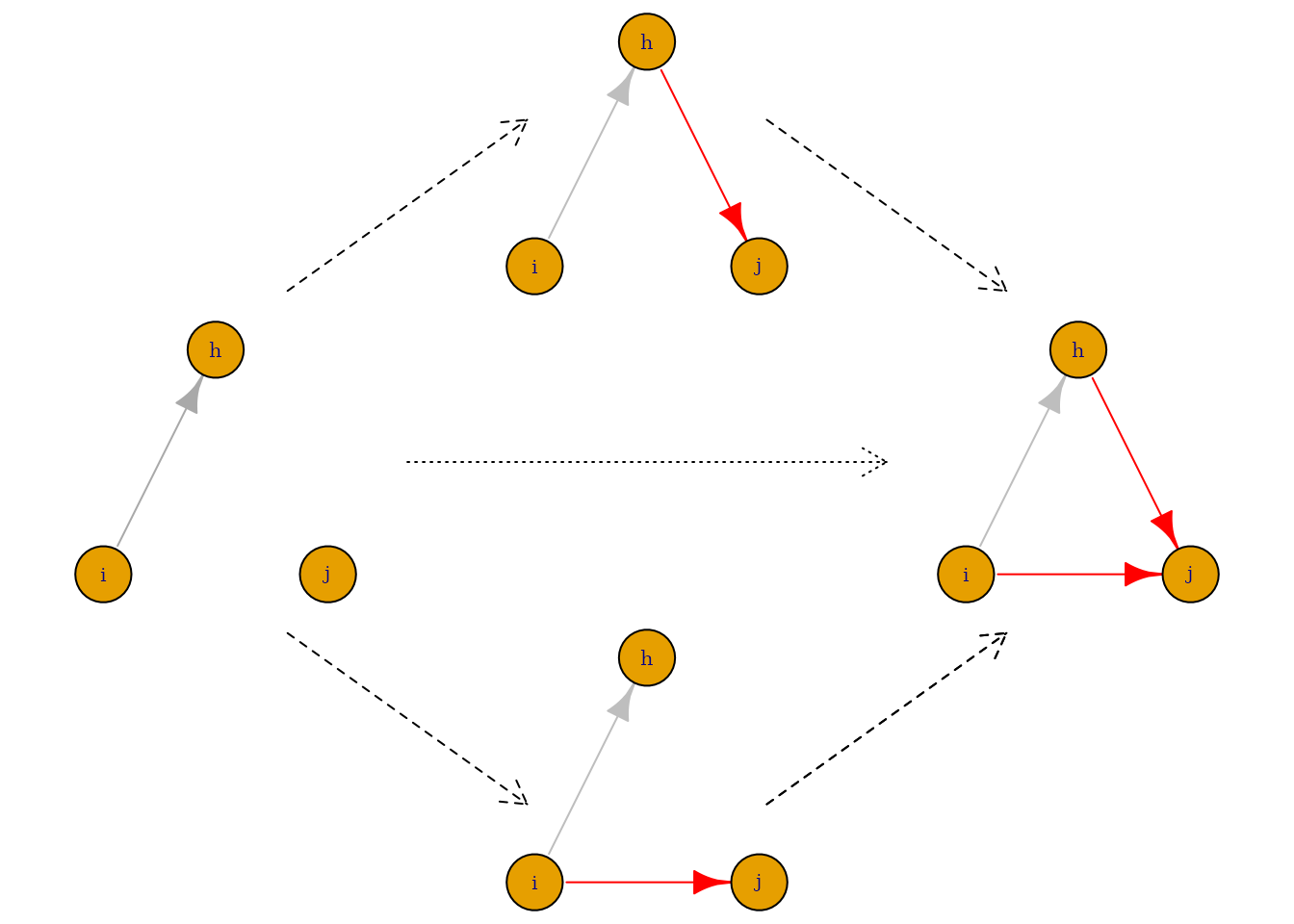
Figure 3.4. Twostep versus ministeps
note: Dashed arrows represent ministeps (long dash) or twostep (short dash); solid arrows represent initial ties (grey) or created ties (red).
3.5. Evaluation function
We start by letting each involved agent evaluate all possible
networks based on the individual evaluation functions. Thus agent1 gives
an evaluation and agent2 gives an evaluation.
Next we have to decide how to combine the separate evaluations of the
two agents. For now, in RsienaTwoStep
, we simply take the
mean of the two separate evaluations as the final evaluation score.
3.6. Choice function
Here we follow the same logic as before. If we know the evaluation score of each network we simply apply a Mc Fadden’s choice function. That is, the actors together ‘decide’ on the future network out of the possible alternative networks in the choice set, given the combined evaluation of these networks. Thus we see the dyad formed by agent1 and agent2 as the decision agent. Please note that agents (or rather the dyad) thus favor the network with the highest combined ‘utility’ score. This is not necessarily the network that would give one of the two agents the highest satisfaction.
3.7. Stopping rule
Once again the logic is exactly similar. However, we count a twostep as two ministeps. Thus, if each actor is allowed to make on average 8 ministeps, actors are allowed to make on average 4 twosteps.
3.8. Example
ts_sims(startvalues = c(2,-1,2), net1=ts_net2, statistics=list(ts_degree, ts_recip),nsims=1, p2step=c(0,1,0), chain = TRUE )
#> [1] "nsim: 1"
#> [[1]]
#> [[1]]$final
#> [[1]]$final$net_n
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 1 1 1
#> [3,] 0 1 0 0 0
#> [4,] 0 1 1 0 0
#> [5,] 0 0 0 0 0
#>
#>
#> [[1]]$chain
#> [[1]]$chain$nets
#> [[1]]$chain$nets[[1]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 1 1
#> [3,] 1 0 0 0 0
#> [4,] 0 1 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[2]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 1 1
#> [3,] 0 0 0 0 0
#> [4,] 0 1 0 0 0
#> [5,] 0 0 0 1 0
#>
#> [[1]]$chain$nets[[3]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 1
#> [2,] 0 0 0 1 1
#> [3,] 0 0 0 0 0
#> [4,] 0 1 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[4]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 1 1
#> [3,] 0 0 0 0 0
#> [4,] 0 1 1 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[5]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 1 1 1
#> [3,] 0 1 0 0 0
#> [4,] 0 1 1 0 0
#> [5,] 0 0 0 0 0
4. ABM simstep
4.1. Logic
A second way in which the ministep assumption can be relaxed is to allow the same actor to make two ministeps simultaneously. Suppose a triad formed by actors i, j and h and a tie between h and j.
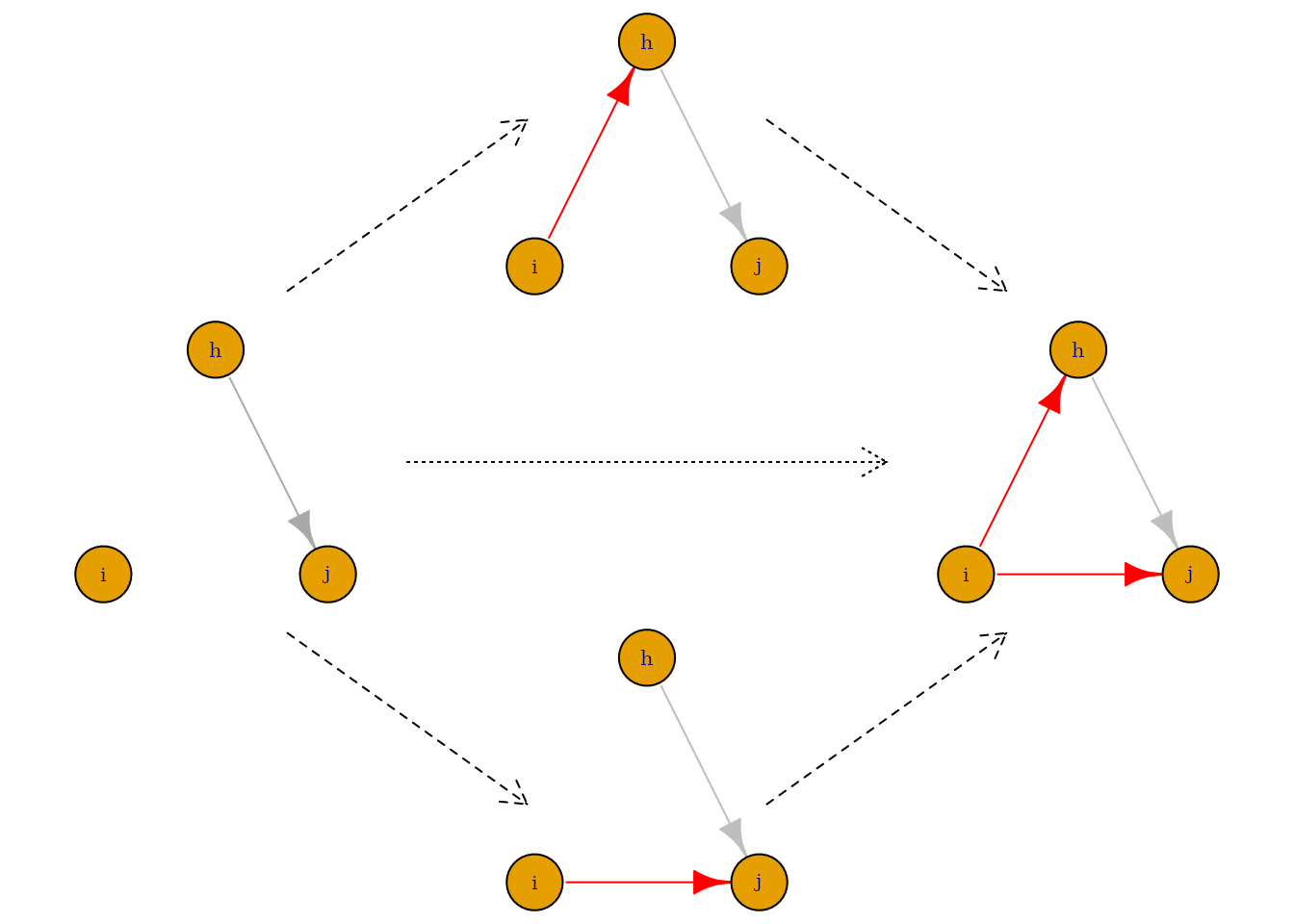
Figure 4.1. Simstep versus ministeps
note: Dashed arrows represent ministeps (long dash) or simstep (short dash); solid arrows represent initial ties (grey) or created ties (red).
Suppose, actors evaluate transitive triplet structures positively.
Normally, under the ministep assumption, actor i should first
make a tie to actor h (or j) and only when it is its
turn again to make a tie change, make a tie to actor j (or
h). Naturally, in larger networks a lot could have happened in
the mean time. Given the network structure at the time, actor i
is allowed to make the second ministep, actor i may not favor
making the additional tie to actor j (or h)
anymore.
Also, actor i may not even want to create a tie to actor
h or j if it is not already sure it can close the
triad immediately afterwards (or even simultaneously). Naturally, in
real life situations, it is not that strange to create multiple ties at
(almost) the same time. See for example the paper on the impact of Kudos
on running behavior (Franken, Bekhuis, and Tolsma
2023). If you are on Strava (or on any other social media for
that matter), it is very common to give multiple kudos (or likes) to
different people in your network at - more or less - the same time.
4.2. Possible networks after simstep
Simply all networks that could arise after two sequential ministeps made by one actor (including the no change option). Please note that the choice set only contains unique network configurations.
4.3. Network statistics
We use the same network statistics as for the ABM ministep. But
please note, that not all existing network statistics of
RSiena
make sense. See for example Figure 4.1. above.
Suppose the evaluation of the transitive triad for actor i
depends on whether path i to j was the closing path or
the path i to h. In a simstep, both ties may be
created simultaneously and we cannot distinguish these two routes.
4.6. Stopping rule
Once again the logic is exactly similar. However, we count a simstep as two ministeps. Thus if each actor is allowed to make on average 8 ministeps, actors are allowed to make on average 4 simsteps.
4.7. Example
ts_sims(startvalues = c(2,-2,2,2), net1=ts_net2, statistics=list(ts_degree, ts_recip, ts_outAct),nsims=1, p2step=c(0,0,1), chain = TRUE )
#> [1] "nsim: 1"
#> [[1]]
#> [[1]]$final
#> [[1]]$final$net_n
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 1 0 1 1 1
#> [3,] 1 1 0 1 0
#> [4,] 0 0 0 0 0
#> [5,] 0 1 1 0 0
#>
#>
#> [[1]]$chain
#> [[1]]$chain$nets
#> [[1]]$chain$nets[[1]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 0 0 0 0 1
#> [3,] 1 1 0 1 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[2]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 1 0 1 0 1
#> [3,] 1 1 0 1 0
#> [4,] 0 0 0 0 0
#> [5,] 0 0 0 0 0
#>
#> [[1]]$chain$nets[[3]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 1 0 1 0 1
#> [3,] 1 1 0 1 0
#> [4,] 0 0 0 0 0
#> [5,] 0 1 1 0 0
#>
#> [[1]]$chain$nets[[4]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 1 0 1 1 1
#> [3,] 1 1 0 1 0
#> [4,] 0 0 0 0 0
#> [5,] 0 1 1 0 0
#>
#> [[1]]$chain$nets[[5]]
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 0 0 0 0 0
#> [2,] 1 0 1 1 1
#> [3,] 1 1 0 1 0
#> [4,] 0 0 0 0 0
#> [5,] 0 1 1 0 0
5. Network census
5.1. Simulate networks
Let us simulate 100 times the outcome of a ABM twostep process and only save the final network
5.2. Dyad and triad configurations
Now we want to count the dyad and triad configurations.
df_dyads <- ts_dyads(nets, forplot = FALSE, simtype="twostep: random")
df_triads <- ts_triads(nets, forplot = FALSE, simtype="twostep: random")
df_dyads
df_triads
#> Mut Asym Null type
#> 1 5 2 3 twostep: random
#> 2 4 4 2 twostep: random
#> 3 3 6 1 twostep: random
#> 4 4 4 2 twostep: random
#> 5 4 4 2 twostep: random
#> 6 4 4 2 twostep: random
#> 7 4 2 4 twostep: random
#> 8 5 2 3 twostep: random
#> 9 4 4 2 twostep: random
#> 10 5 2 3 twostep: random
#> 11 5 2 3 twostep: random
#> 12 4 4 2 twostep: random
#> 13 3 6 1 twostep: random
#> 14 4 4 2 twostep: random
#> 15 5 2 3 twostep: random
#> 16 6 0 4 twostep: random
#> 17 4 4 2 twostep: random
#> 18 5 2 3 twostep: random
#> 19 4 3 3 twostep: random
#> 20 2 7 1 twostep: random
#> 21 4 4 2 twostep: random
#> 22 4 3 3 twostep: random
#> 23 4 4 2 twostep: random
#> 24 4 4 2 twostep: random
#> 25 5 2 3 twostep: random
#> 26 5 2 3 twostep: random
#> 27 5 2 3 twostep: random
#> 28 6 0 4 twostep: random
#> 29 5 2 3 twostep: random
#> 30 4 2 4 twostep: random
#> 31 4 3 3 twostep: random
#> 32 4 3 3 twostep: random
#> 33 4 4 2 twostep: random
#> 34 4 4 2 twostep: random
#> 35 5 2 3 twostep: random
#> 36 4 3 3 twostep: random
#> 37 4 4 2 twostep: random
#> 38 5 2 3 twostep: random
#> 39 5 2 3 twostep: random
#> 40 5 1 4 twostep: random
#> 41 5 1 4 twostep: random
#> 42 3 5 2 twostep: random
#> 43 4 3 3 twostep: random
#> 44 6 0 4 twostep: random
#> 45 5 2 3 twostep: random
#> 46 3 6 1 twostep: random
#> 47 4 4 2 twostep: random
#> 48 5 2 3 twostep: random
#> 49 4 4 2 twostep: random
#> 50 4 4 2 twostep: random
#> 51 4 4 2 twostep: random
#> 52 4 4 2 twostep: random
#> 53 4 3 3 twostep: random
#> 54 3 6 1 twostep: random
#> 55 5 2 3 twostep: random
#> 56 5 2 3 twostep: random
#> 57 6 0 4 twostep: random
#> 58 3 5 2 twostep: random
#> 59 5 2 3 twostep: random
#> 60 5 2 3 twostep: random
#> 61 4 3 3 twostep: random
#> 62 4 4 2 twostep: random
#> 63 3 6 1 twostep: random
#> 64 5 2 3 twostep: random
#> 65 5 2 3 twostep: random
#> 66 6 0 4 twostep: random
#> 67 4 3 3 twostep: random
#> 68 5 2 3 twostep: random
#> 69 5 2 3 twostep: random
#> 70 5 2 3 twostep: random
#> 71 5 2 3 twostep: random
#> 72 4 3 3 twostep: random
#> 73 4 3 3 twostep: random
#> 74 4 2 4 twostep: random
#> 75 6 0 4 twostep: random
#> 76 4 4 2 twostep: random
#> 77 4 4 2 twostep: random
#> 78 5 2 3 twostep: random
#> 79 4 4 2 twostep: random
#> 80 5 2 3 twostep: random
#> 81 4 3 3 twostep: random
#> 82 5 2 3 twostep: random
#> 83 3 6 1 twostep: random
#> 84 5 2 3 twostep: random
#> 85 5 2 3 twostep: random
#> 86 5 2 3 twostep: random
#> 87 4 4 2 twostep: random
#> 88 5 2 3 twostep: random
#> 89 5 2 3 twostep: random
#> 90 4 4 2 twostep: random
#> 91 4 3 3 twostep: random
#> 92 5 2 3 twostep: random
#> 93 5 2 3 twostep: random
#> 94 5 2 3 twostep: random
#> 95 4 3 3 twostep: random
#> 96 5 2 3 twostep: random
#> 97 4 3 3 twostep: random
#> 98 5 2 3 twostep: random
#> 99 5 2 3 twostep: random
#> 100 4 4 2 twostep: random
#> 003 012 102 021D 021U 021C 111D 111U 030T 030C 201 120D 120U 120C 210 300
#> 1 0 1 1 0 0 0 0 2 0 0 3 0 0 0 3 0
#> 2 0 0 0 0 1 0 1 2 1 0 2 0 0 1 2 0
#> 3 0 0 0 2 0 0 0 1 2 0 0 0 2 0 3 0
#> 4 0 0 0 0 1 1 0 2 0 0 2 0 2 0 2 0
#> 5 0 1 0 1 0 0 0 2 0 0 1 0 3 0 1 1
#> 6 0 1 0 0 0 0 0 3 0 0 1 1 1 1 2 0
#> 7 1 1 1 0 0 0 0 3 0 0 2 0 1 0 0 1
#> 8 0 1 2 0 0 0 0 1 0 0 2 0 1 0 2 1
#> 9 0 0 0 0 0 0 2 4 0 0 0 1 1 1 0 1
#> 10 1 0 0 0 0 0 0 4 0 0 2 0 0 0 2 1
#> 11 0 1 2 0 0 0 0 3 0 0 0 0 0 0 2 2
#> 12 0 0 1 0 0 2 0 1 0 0 1 0 1 1 3 0
#> 13 0 0 0 0 0 1 1 0 2 0 1 0 2 2 1 0
#> 14 0 0 0 0 0 2 1 2 0 0 1 0 1 1 1 1
#> 15 0 0 2 0 0 0 3 1 0 0 1 0 0 0 2 1
#> 16 0 0 3 0 0 0 0 0 0 0 6 0 0 0 0 1
#> 17 0 0 0 0 0 1 1 2 1 0 2 0 0 1 2 0
#> 18 0 1 1 0 0 0 0 3 0 0 2 0 1 0 0 2
#> 19 0 1 1 0 0 1 2 0 0 0 2 0 1 0 2 0
#> 20 0 0 0 1 0 1 1 0 2 1 0 0 2 1 1 0
#> 21 0 0 0 0 0 0 2 4 1 0 0 0 0 0 3 0
#> 22 0 1 0 0 0 0 2 3 0 0 2 0 1 0 1 0
#> 23 0 1 0 1 0 0 0 2 0 0 1 0 1 1 3 0
#> 24 0 0 1 0 1 0 1 2 1 0 0 0 1 0 2 1
#> 25 0 0 1 0 0 0 2 2 0 0 3 0 0 0 2 0
#> 26 0 1 0 0 0 0 1 1 0 0 5 0 1 0 1 0
#> 27 0 0 2 0 0 0 1 2 0 0 2 0 1 0 1 1
#> 28 1 0 2 0 0 0 0 0 0 0 5 0 0 0 0 2
#> 29 0 0 2 0 0 1 1 1 0 0 2 0 0 0 2 1
#> 30 1 1 1 0 0 0 0 4 0 0 1 0 0 0 1 1
#> 31 1 0 0 1 0 0 0 4 0 0 1 0 1 0 1 1
#> 32 0 1 1 1 0 0 0 3 0 0 1 0 1 0 1 1
#> 33 0 0 0 0 1 1 1 2 0 0 1 1 0 1 1 1
#> 34 0 1 0 0 0 0 0 3 1 0 1 0 2 0 1 1
#> 35 0 0 1 0 0 0 2 3 0 0 2 0 0 0 1 1
#> 36 0 1 1 0 0 1 0 2 0 0 2 0 1 1 0 1
#> 37 0 0 1 0 0 1 0 3 1 0 0 0 1 0 2 1
#> 38 0 0 2 1 0 0 0 2 0 0 2 0 0 0 2 1
#> 39 0 2 0 0 0 0 1 1 0 0 3 0 0 0 2 1
#> 40 1 0 2 0 0 0 0 2 0 0 3 0 0 0 1 1
#> 41 1 0 2 0 0 0 0 2 0 0 3 0 0 0 1 1
#> 42 0 0 0 2 2 0 0 1 0 0 1 0 2 0 2 0
#> 43 0 2 0 0 0 0 1 2 0 0 2 0 0 1 2 0
#> 44 0 0 4 0 0 0 0 0 0 0 4 0 0 0 0 2
#> 45 0 1 2 0 0 0 0 1 0 0 2 0 0 1 2 1
#> 46 0 0 0 0 1 0 0 2 2 0 0 0 4 0 0 1
#> 47 0 1 0 0 0 0 0 3 1 0 1 0 2 0 1 1
#> 48 0 2 1 0 0 0 0 0 0 0 3 0 0 1 2 1
#> 49 0 0 0 0 0 0 2 4 0 0 0 1 2 0 0 1
#> 50 0 0 1 1 0 0 2 1 0 0 0 0 1 1 3 0
#> 51 0 0 0 0 1 0 2 2 0 0 1 1 1 0 2 0
#> 52 0 0 1 1 0 1 0 2 0 0 0 0 1 0 4 0
#> 53 0 2 0 0 0 0 0 4 0 0 1 0 1 0 1 1
#> 54 0 0 0 1 0 1 0 1 1 0 0 0 3 1 2 0
#> 55 0 0 1 0 0 1 2 2 0 0 2 0 0 0 0 2
#> 56 0 1 1 0 0 0 1 2 0 0 2 0 0 1 0 2
#> 57 1 0 2 0 0 0 0 0 0 0 5 0 0 0 0 2
#> 58 0 1 0 0 2 0 0 1 1 0 1 0 1 1 2 0
#> 59 0 1 0 0 0 0 2 1 0 0 4 0 0 0 2 0
#> 60 0 0 2 0 1 0 1 0 0 0 3 0 0 0 3 0
#> 61 1 0 0 0 0 0 2 2 0 0 2 1 1 0 1 0
#> 62 0 0 0 0 1 0 0 4 1 0 1 0 1 0 1 1
#> 63 0 0 0 0 1 0 1 0 2 0 1 0 3 1 1 0
#> 64 0 0 2 0 0 0 1 3 0 0 1 0 0 0 2 1
#> 65 0 1 1 0 0 0 1 1 0 0 3 0 1 0 1 1
#> 66 0 0 4 0 0 0 0 0 0 0 4 0 0 0 0 2
#> 67 0 2 0 0 0 0 0 4 0 0 1 0 1 0 1 1
#> 68 0 1 1 0 1 0 0 1 0 0 3 0 0 0 2 1
#> 69 0 1 1 0 0 1 0 1 0 0 3 0 0 0 2 1
#> 70 0 1 0 0 0 0 1 2 0 0 4 0 0 0 2 0
#> 71 0 1 1 1 0 0 1 0 0 0 3 0 0 0 2 1
#> 72 0 1 0 1 0 0 2 1 0 0 3 0 0 1 1 0
#> 73 0 0 3 1 0 1 0 1 0 0 0 0 0 1 2 1
#> 74 1 0 2 1 0 0 0 3 0 0 1 0 0 0 1 1
#> 75 0 0 4 0 0 0 0 0 0 0 4 0 0 0 0 2
#> 76 0 0 0 0 0 2 1 2 0 0 1 0 1 0 3 0
#> 77 0 0 1 1 0 1 0 2 0 0 0 0 1 0 4 0
#> 78 0 2 1 0 0 0 0 0 0 0 3 0 1 0 2 1
#> 79 0 1 0 1 0 0 0 2 0 0 1 0 3 0 1 1
#> 80 0 1 1 0 0 0 0 2 0 0 3 0 0 0 3 0
#> 81 0 1 1 0 0 1 1 2 0 0 1 0 0 0 3 0
#> 82 0 1 0 0 0 0 1 1 0 0 5 0 1 0 1 0
#> 83 0 0 0 1 0 0 1 1 1 0 0 2 3 0 1 0
#> 84 0 1 0 0 0 0 1 1 0 0 5 1 0 0 1 0
#> 85 0 1 1 0 0 0 0 3 0 0 2 0 1 0 0 2
#> 86 1 0 0 0 0 0 0 4 0 0 2 0 0 0 2 1
#> 87 0 1 0 1 0 0 0 1 0 0 2 0 1 2 2 0
#> 88 0 1 1 0 0 0 0 3 0 0 2 0 0 0 2 1
#> 89 1 0 0 0 0 0 0 4 0 0 2 0 0 0 2 1
#> 90 0 0 1 2 0 0 0 1 0 0 1 1 1 0 3 0
#> 91 0 0 2 0 0 0 1 3 1 0 1 0 0 0 2 0
#> 92 0 1 2 0 0 0 0 1 0 0 2 0 1 0 2 1
#> 93 1 0 0 0 0 0 0 4 0 0 2 0 1 0 0 2
#> 94 0 0 2 0 0 1 0 1 0 0 3 0 0 0 3 0
#> 95 0 2 0 0 0 0 0 4 0 0 1 0 1 0 1 1
#> 96 0 1 1 0 0 0 0 3 0 0 2 0 0 0 2 1
#> 97 0 1 1 0 0 1 0 3 0 0 1 0 1 0 1 1
#> 98 1 0 0 0 0 0 0 4 0 0 2 0 1 0 0 2
#> 99 0 1 1 0 0 0 0 3 0 0 2 0 0 0 2 1
#> 100 0 1 0 0 0 0 0 2 0 0 2 1 1 2 1 0
#> type
#> 1 twostep: random
#> 2 twostep: random
#> 3 twostep: random
#> 4 twostep: random
#> 5 twostep: random
#> 6 twostep: random
#> 7 twostep: random
#> 8 twostep: random
#> 9 twostep: random
#> 10 twostep: random
#> 11 twostep: random
#> 12 twostep: random
#> 13 twostep: random
#> 14 twostep: random
#> 15 twostep: random
#> 16 twostep: random
#> 17 twostep: random
#> 18 twostep: random
#> 19 twostep: random
#> 20 twostep: random
#> 21 twostep: random
#> 22 twostep: random
#> 23 twostep: random
#> 24 twostep: random
#> 25 twostep: random
#> 26 twostep: random
#> 27 twostep: random
#> 28 twostep: random
#> 29 twostep: random
#> 30 twostep: random
#> 31 twostep: random
#> 32 twostep: random
#> 33 twostep: random
#> 34 twostep: random
#> 35 twostep: random
#> 36 twostep: random
#> 37 twostep: random
#> 38 twostep: random
#> 39 twostep: random
#> 40 twostep: random
#> 41 twostep: random
#> 42 twostep: random
#> 43 twostep: random
#> 44 twostep: random
#> 45 twostep: random
#> 46 twostep: random
#> 47 twostep: random
#> 48 twostep: random
#> 49 twostep: random
#> 50 twostep: random
#> 51 twostep: random
#> 52 twostep: random
#> 53 twostep: random
#> 54 twostep: random
#> 55 twostep: random
#> 56 twostep: random
#> 57 twostep: random
#> 58 twostep: random
#> 59 twostep: random
#> 60 twostep: random
#> 61 twostep: random
#> 62 twostep: random
#> 63 twostep: random
#> 64 twostep: random
#> 65 twostep: random
#> 66 twostep: random
#> 67 twostep: random
#> 68 twostep: random
#> 69 twostep: random
#> 70 twostep: random
#> 71 twostep: random
#> 72 twostep: random
#> 73 twostep: random
#> 74 twostep: random
#> 75 twostep: random
#> 76 twostep: random
#> 77 twostep: random
#> 78 twostep: random
#> 79 twostep: random
#> 80 twostep: random
#> 81 twostep: random
#> 82 twostep: random
#> 83 twostep: random
#> 84 twostep: random
#> 85 twostep: random
#> 86 twostep: random
#> 87 twostep: random
#> 88 twostep: random
#> 89 twostep: random
#> 90 twostep: random
#> 91 twostep: random
#> 92 twostep: random
#> 93 twostep: random
#> 94 twostep: random
#> 95 twostep: random
#> 96 twostep: random
#> 97 twostep: random
#> 98 twostep: random
#> 99 twostep: random
#> 100 twostep: random
5.3. Violin plots
If you want to make violin plots of these census, it is best to set
the option forplot
to TRUE
.
Just as an example:
df_dyads2 <- ts_dyads(nets, forplot = TRUE, simtype="twostep: random")
library(ggplot2)
p <- ggplot(df_dyads2, aes(x=x, y=y, fill=type)) +
geom_violin(position=position_dodge(1)) +
stat_summary(fun = mean,
geom = "errorbar",
fun.max = function(x) mean(x) + sd(x),
fun.min = function(x) mean(x) - sd(x),
width=.1,
color="red", position=position_dodge(1)) +
stat_summary(fun = mean,
geom = "point",
color="red", position=position_dodge(1)) +
xlab('dyad configuration') + ylab('count')
p
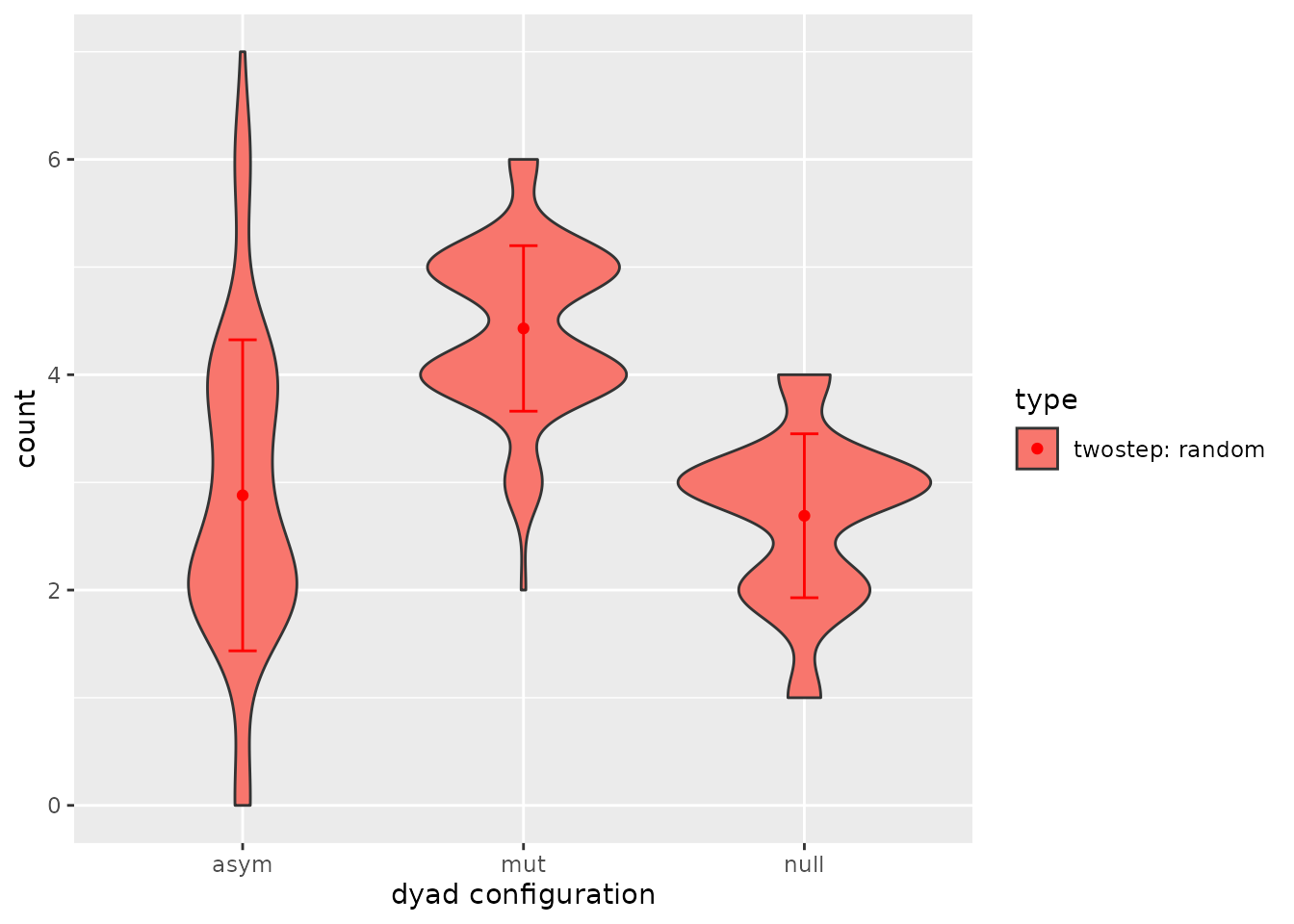
Figure 5.3. Dyad census